若您覺得文章寫得不錯,請點選文章上的廣告,來支持小編,謝謝。
If you like this post, please click the ads on the blog or buy me a coffee. Thank you very much.
練習一:入學許可
如果您的在校成績有高於80分,就可以申請自己第一志願的大學。現在請設計一程式可讀取使用者的在校成績,若使用者可以申請第一志願的大學就輸出「YES」,否則輸出「NO」。
如果您的在校成績有高於80分,就可以申請自己第一志願的大學。現在請設計一程式可讀取使用者的在校成績,若使用者可以申請第一志願的大學就輸出「YES」,否則輸出「NO」。
Exercise 1: College Admission
If you got GPA higher than 4.0 in transcript then you can apply for your first choice. Now write a C program read GPA from the keyboard and print "YES" if you can apply otherwise print "NO".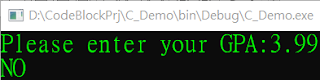
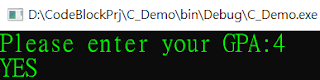
練習二:APCS成績級分
撰寫一個可輸入APCS成績的C語言程式,此程式會依據下表輸出對應的級分。
練習六參考解法一:
Exercise 6 solution 1:
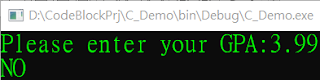
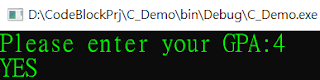
練習一參考解法:
Exercise 1 solution:
Exercise 1 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | #include <stdio.h> #include <stdlib.h> int main() { float gpa; printf("Please enter your GPA:"); scanf("%f", &gpa); if(gpa < 4.0) printf("NO\n"); else printf("YES\n"); return 0; } |
練習二:APCS成績級分
撰寫一個可輸入APCS成績的C語言程式,此程式會依據下表輸出對應的級分。
級分 |
分數範圍 |
五 |
90~100 |
四 |
70~89 |
三 |
50~69 |
二 |
30~49 |
一 |
0~29 |
Exercise 2: C Programming GPA
Write a C program that gets C Programming score from the user. Then print the grade depending on the given scale(See the following table).


Grade |
Scale |
A |
90~100 |
B |
70~89 |
C |
50~69 |
D |
30~49 |
F |
0~29 |


練習二參考解法:
Exercise 2 solution:
練習三:成年了嗎?
每個國家都會規定幾歲以上才是成年,那我們就來寫個程式來判斷年齡是否有成年吧。此練習題是18歲以上算成年喔!成年後就可以做好多事啊!
Exercise 2 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #include <stdio.h> #include <stdlib.h> int main() { int apcsScore; printf("Enter your C programming score:"); scanf("%d", &apcsScore); printf("Grade:"); if(apcsScore >= 90) printf("A"); else if(apcsScore >= 70) printf("B"); else if(apcsScore >= 50) printf("C"); else if(apcsScore >= 30) printf("D"); else if(apcsScore >= 0) printf("F"); printf(".\n"); return 0; } |
練習三:成年了嗎?
每個國家都會規定幾歲以上才是成年,那我們就來寫個程式來判斷年齡是否有成年吧。此練習題是18歲以上算成年喔!成年後就可以做好多事啊!
Exercise 3: Are you an adult?
In some countries, men and women come of age at 18. Now let's write a C program to determine is someone an adult. There are many things that adults can do.
練習三參考解法:
Exercise 3 solution:
練習四:奇數或偶數
設計一個可以判斷一個整數是奇數或是偶數的程式。
練習三參考解法:
Exercise 3 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> #include <stdlib.h> int main() { int age; printf("Enter your age:"); scanf("%d", &age); if(age >= 18) printf("Congratulation!\n"); else printf("There are %d years left to be an adult.\n", 18 - age); return 0; } |
設計一個可以判斷一個整數是奇數或是偶數的程式。
Exercise 4: Odd or even
Exercise 4 solution:
練習五:可整除嗎?
寫一個可以判斷一個數是否可以同時被 3 與 11 整除。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | #include <stdio.h> #include <stdlib.h> int main() { int number; printf("Please input an integer:"); scanf("%d", &number); if( number % 2 ) printf("%d is odd.\n", number); else printf("%d is even.\n", number); return 0; } |
練習五:可整除嗎?
寫一個可以判斷一個數是否可以同時被 3 與 11 整除。
Exercise 5: Divisible
Take an integer from user and determine whether it is divisible by 3 and 11.
練習五參考解法:
Exercise 5 solution:
練習六:最大值
輸入三個整數,程式輸出最大值。
Take an integer from user and determine whether it is divisible by 3 and 11.
練習五參考解法:
Exercise 5 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | #include <stdio.h> #include <stdlib.h> int main() { int number; printf("Please input an integer:"); scanf("%d", &number); if( number % 3 == 0 && number % 11 == 0 ) printf("%d is divisible by 3 and 11.\n", number); else printf("%d isn't divisible by 3 and 11.\n", number); return 0; } |
練習六:最大值
輸入三個整數,程式輸出最大值。
Exercise 6 solution 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #include <stdio.h> #include <stdlib.h> int main() { int a, b, c; printf("Enter three integer:"); scanf("%d%d%d", &a, &b, &c); printf("From %d, %d, %d, the maximum is ", a, b, c); if(a > b && a > c) printf("%d.\n", a); else if(b > c) printf("%d.\n", b); else printf("%d.\n", c); return 0; } |
練習六參考解法二:
Exercise 6 solution 2:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include <stdio.h> #include <stdlib.h> int main() { int a, b, c; printf("Enter three integer:"); scanf("%d%d%d", &a, &b, &c); int max; if(a > b) max = a; else max = b; if(max < c) max = c; printf("From %d, %d, %d, the maximum is %d.\n", a, b, c, max); return 0; } |
練習七:母音?子音?
設計一個程式可以判斷英文字母是母音或子音。
設計一個程式可以判斷英文字母是母音或子音。
練習七參考解法:
Exercise 7 solution:
Exercise 7 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #include <stdio.h> #include <stdlib.h> int main() { char letter; printf("Enter a letter:"); scanf(" %c", &letter); if( letter == 'a' || letter == 'A' || letter == 'e' || letter == 'E' || letter == 'i' || letter == 'I' || letter == 'o' || letter == 'O' || letter == 'u' || letter == 'U' ) printf("%c is Vowel.\n", letter); else printf("%c is Consonant.\n", letter); return 0; } |
練習八:月份英文名稱
設計一程式可讀取 [1 - 12] 的整數,輸出對應月份的英文名稱。
設計一程式可讀取 [1 - 12] 的整數,輸出對應月份的英文名稱。
Exercise 8: Months Name
Write a C program that gets an integer [1 - 12] from user. And print the corresponding month name.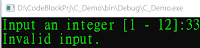
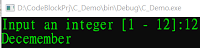
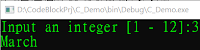
練習八參考解法:
Exercise 8 solution:
Write a C program that gets an integer [1 - 12] from user. And print the corresponding month name.
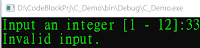
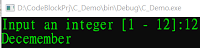
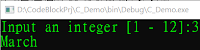
練習八參考解法:
Exercise 8 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | #include <stdio.h> #include <stdlib.h> int main() { int m; printf("Input an integer [1 - 12]:"); scanf("%d", &m); if(m == 1) { printf("January\n"); } else if(m == 2) { printf("February\n"); } else if(m == 3) { printf("March\n"); } else if(m == 4) { printf("April\n"); } else if(m == 5) { printf("May\n"); } else if(m == 6) { printf("June\n"); } else if(m == 7) { printf("July\n"); } else if(m == 8) { printf("August\n"); } else if(m == 9) { printf("September\n"); } else if(m == 10) { printf("October\n"); } else if(m == 11) { printf("November\n"); } else if(m == 12) { printf("Decemember\n"); } else { printf("Invalid input.\n"); } return 0; } |
練習九:第幾象限
設計一個可以判斷(x, y)座標在第幾象限的程式。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | #include <stdio.h> #include <stdlib.h> int main() { int x, y; printf("Enter the value of (X Y):"); scanf("%d%d", &x, &y); if(x > 0 && y > 0) { printf("First"); } else if(x < 0 && y > 0) { printf("Second"); } else if(x < 0 && y < 0) { printf("Third"); } else if(x > 0 && y < 0) { printf("Fourth"); } printf(" Quadrant.\n"); return 0; } |
沒有留言:
張貼留言