若您覺得文章寫得不錯,請點選文章上的廣告,來支持小編,謝謝。
If you like this post, please click the ads on the blog or buy me a coffee. Thank you very much.
練習一:奇數數列
使用迴圈輸出 1 到 100 之間的奇數。
使用迴圈輸出 1 到 100 之間的奇數。
Exercise 1: Odd Sequence
練習二:範圍
輸出 300 到 500 之間可以被 3 與 11 整除的整數。
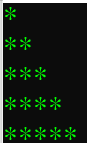
練習三參考解法:
Exercise 3 solution:
練習四:乘法表
輸入一個整數,程式輸出此整數從 1 乘到 10 的結果,例如輸入整數 7 時,輸出結果會是
練習四參考解法:
Exercise 4 solution:
練習五:等差數列加總
設計一程式可算出底下等差數列的總和。
Exercise 7: Star Triangle 2
Design a C program that print the following star triangle.
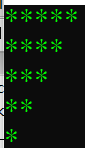
練習七參考解法:
Exercise 7 solution:
Print the odd numbers between 1 to 100.
練習一參考解法:
Exercise 1 solution:
練習一參考解法:
Exercise 1 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | #include <stdio.h> #include <stdlib.h> int main() { int i; // FOR loop 1 for(i = 1; i <= 100; i += 2) printf("%d, ", i); printf("\n\n"); // FOR loop 2 for(i = 1; i <= 100; i++) if(i % 2) printf("%d, ", i); printf("\n\n"); // WHILE loop i = 1; while( i <= 100) { if(i % 2) printf("%d, ", i); i++; } printf("\n\n"); // DO-WHILE loop i = 1; do { if(i % 2) printf("%d, ", i); i++; } while(i <= 100); return 0; } |
練習二:範圍
輸出 300 到 500 之間可以被 3 與 11 整除的整數。
Exercise 2: Range
Print the numbers between 300 to 500 which are divisible by 3 and 11.
練習二參考解法:
Exercise 2 solution:
練習三:星星三角形
設計一程式,輸出底下的星星三角形。
Print the numbers between 300 to 500 which are divisible by 3 and 11.
練習二參考解法:
Exercise 2 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | #include <stdio.h> #include <stdlib.h> int main() { int i; // FOR loop for(i = 300; i <= 500; i++) if(i % 3 == 0 && i % 11 == 0) printf("%d, ", i); printf("\n\n"); // WHILE loop i = 300; while( i <= 500) { if(i % 3 == 0 && i % 11 == 0) printf("%d, ", i); i++; } printf("\n\n"); // DO-WHILE loop i = 300; do { if(i % 3 == 0 && i % 11 == 0) printf("%d, ", i); i++; } while(i <= 500); return 0; } |
練習三:星星三角形
設計一程式,輸出底下的星星三角形。
Exercise 3: Star Triangle
Design a C program that print the star triangle.
Design a C program that print the star triangle.
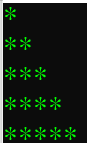
練習三參考解法:
Exercise 3 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> int main() { int i, j; for(i = 0; i <= 4; i++) { for(j = 0; j <= i; j++) { printf("*"); } printf("\n"); } return 0; } |
練習四:乘法表
輸入一個整數,程式輸出此整數從 1 乘到 10 的結果,例如輸入整數 7 時,輸出結果會是
7 * 1 = 7
7 * 2 = 14
7 * 3 = 21
.
.
.
7 * 10 = 17
Exercise 4: Multiplication table
Read an integer from user and print it's multiplication table up to 10. For example, if user give 7, the output will be look like the following:
7 * 1 = 7
7 * 2 = 14
7 * 3 = 21
.
.
.
7 * 10 = 17
練習四參考解法:
Exercise 4 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> #include <stdlib.h> int main() { int num; printf("Enter an integer:"); scanf("%d", &num); for(int i = 1; i <= 10; i++) printf("%d * %d = %d\n", num, i, (num*i)); return 0; } |
練習五:等差數列加總
設計一程式可算出底下等差數列的總和。
1 + 4 + 7 + 10 + 13 + ... + 97 + 100
Exercise 5: Sum of an Arithmetic Series
練習五參考解法:
Exercise 5 solution:
練習六:等比數列加總
Write a C program to print the summation of the following series:
1 + 4 + 7 + 10 + 13 + ... + 97 + 100
Exercise 5 solution:
1 2 3 4 5 6 7 8 9 10 11 12 | #include <stdio.h> #include <stdlib.h> int main() { int n, sum = 0; for(n = 1; n <= 100; n += 3) sum = sum + n; printf("Sum is %d\n", sum); return 0; } |
練習六:等比數列加總
設計一程式可算出底下等比數列的總和。
1 + 2 + 4 + 8 + 16 + ... + 524288 + 1048576Exercise 6: Sum of a Geometric Series
練習六參考解法:
Exercise 6 solution:
Write a C program to print the summation of the following series:
1 + 2 + 4 + 8 + 16 + ... + 524288 + 1048576
練習六參考解法:
Exercise 6 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 | #include <stdio.h> #include <stdlib.h> int main() { long n, sum = 0; for(n = 1; n <= 1048576; n *= 2) sum = sum + n; printf("Sum is %ld\n", sum); return 0; } |
練習七:星星三角形二
設計一程式,輸出底下的圖形。
設計一程式,輸出底下的圖形。
Exercise 7: Star Triangle 2
Design a C program that print the following star triangle.
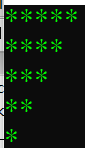
練習七參考解法:
Exercise 7 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> int main() { int i, j; for(i = 0; i <= 4; i++) { for(j = 4; j >= i; j--) { printf("*"); } printf("\n"); } return 0; } |
練習八:英文字母
使用迴圈來輸出英文的大小寫字母。
使用迴圈來輸出英文的大小寫字母。
Exercise 8: Alphabets
Write a C program that uses loop to display English alphabet.
練習八參考解法:
Exercise 8 solution:
Write a C program that uses loop to display English alphabet.
Exercise 8 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #include <stdio.h> #include <stdlib.h> int main() { char letter; printf("Small letters: "); for(letter = 'a'; letter <= 'z'; letter++) printf("%c ", letter); printf("\n\n"); printf("Large letters: "); for(letter = 'A'; letter <= 'Z'; letter++) printf("%c ", letter); return 0; } |
練習九:迴圈與條件判斷
設計一個程式可不斷讓使用者輸入一個整數,直到輸入 0 才結束程式。並判斷此整數是奇數或是偶數。
設計一個程式可不斷讓使用者輸入一個整數,直到輸入 0 才結束程式。並判斷此整數是奇數或是偶數。
Exercise 9: Loop and conditions
Design a C program to determine whether an integer is odd or even repeatedly until 0 is entered.
練習九參考解法:
Exercise 9 solution:
Design a C program to determine whether an integer is odd or even repeatedly until 0 is entered.
練習九參考解法:
Exercise 9 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | #include <stdio.h> #include <stdlib.h> int main() { int number; do { printf("Please input an integer:"); scanf("%d", &number); if( number % 2 ) printf("%d is odd.\n", number); else printf("%d is even.\n", number); }while(number != 0); return 0; } |
練習十:費氏數列
設計可以輸入一個整數 N ,輸出到整數 N 的費氏數列。例如當 N = 22 時,程式輸出:0, 1, 1, 2, 3, 5, 8, 13, 21,
設計可以輸入一個整數 N ,輸出到整數 N 的費氏數列。例如當 N = 22 時,程式輸出:0, 1, 1, 2, 3, 5, 8, 13, 21,
Exercise 10: Fibonacci sequence
Write a C program that gets an integer from user and display Fibonacci numbers up to N. If N is 22, the program should print: 0, 1, 1, 2, 3, 5, 8, 13, 21,
練習十參考解法:
Exercise 10 solution:
Write a C program that gets an integer from user and display Fibonacci numbers up to N. If N is 22, the program should print: 0, 1, 1, 2, 3, 5, 8, 13, 21,
練習十參考解法:
Exercise 10 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | #include <stdio.h> #include <stdlib.h> int main() { int n; printf("Please enter an integer:"); scanf("%d", &n); int first = 0, second = 1, tmp; while(first <= n) { printf("%d, ", first); tmp = first + second; first = second; second = tmp; } return 0; } |
練習十一:數字圖案
設計一程式輸出下圖上的數字圖案。
設計一程式輸出下圖上的數字圖案。
Exercise 11: Number Pattern
Design a C program to display the number pattern in the below picture.
練習十一參考解法:
Exercise 11 solution:
Design a C program to display the number pattern in the below picture.
練習十一參考解法:
Exercise 11 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> #include <stdlib.h> int main() { int n = 9; for(int i = 1; i <= n; i++) { for(int j = 1; j <= i; j++) printf("%d ", i * j); printf("\n"); } return 0; } |
練習十二:質數
設計可以輸入一個整數 N ,輸出到整數 N 的質數數列。例如當 N = 50 時,程式輸出:2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47,
設計可以輸入一個整數 N ,輸出到整數 N 的質數數列。例如當 N = 50 時,程式輸出:2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47,
Exercise 12: Prime
Write a C program that gets an integer from user and display prime numbers up to N. If N is 50, the program should print: 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47,
練習十二參考解法:
Exercise 12 solution:
Write a C program that gets an integer from user and display prime numbers up to N. If N is 50, the program should print: 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47,
練習十二參考解法:
Exercise 12 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | #include <stdio.h> #include <stdlib.h> int main() { int n, isPrime; printf("Enter an integer:"); scanf("%d", &n); for(int p = 2; p <= n; p++) { isPrime = 1; for(int i = 2; i * i <= p; i++) { if(p % i == 0) { isPrime = 0; break; } } if(isPrime == 1) printf("%d, ", p); } return 0; } |
沒有留言:
張貼留言