若您覺得文章寫得不錯,請點選文章上的廣告,來支持小編,謝謝。
If you like this post, please click the ads on the blog or buy me a coffee. Thank you very much.
練習一:加法函數設計一函數可以輸入兩個參數a與b,並回傳 a 加 b的結果。
Exercise 1: Adding function
練習二:整數乘法
設計一函數可以輸入兩個參數a與b,並回傳 a 乘 b的結果。
練習四:歡迎訊息
Design a function that takes two parameters and return the result of a + b.
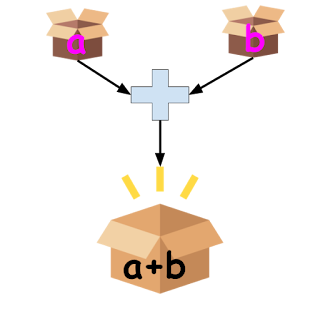
練習一參考解法:
Exercise 1 solution:
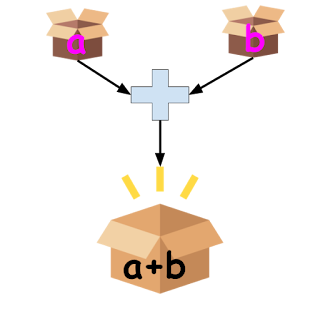
練習一參考解法:
Exercise 1 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> #include <stdlib.h> float add(float a, float b) { return a + b; } int main() { float x = 3.4f, y = 3.6f; printf("%.2f + %.2f = %.2f\n", x, y, add(x, y)); return 0; } |
練習二:整數乘法
設計一函數可以輸入兩個參數a與b,並回傳 a 乘 b的結果。
Exercise 2: Multiplying Two Integers
Design a function that takes two parameters and return the result of a * b.
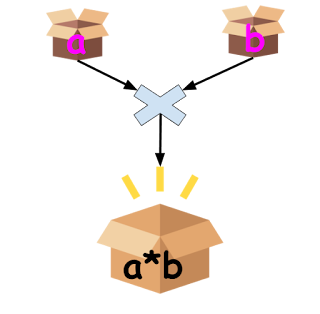
練習二參考解法:
Exercise 2 solution:
練習三:奇數或偶數
Design a function that takes two parameters and return the result of a * b.
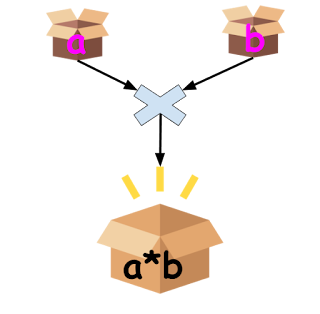
練習二參考解法:
Exercise 2 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> #include <stdlib.h> int multiply(int a, int b) { return a * b; } int main() { int x = 3, y = 4; printf("%3d + %3d = %3d\n", x, y, multiply(x, y)); return 0; } |
練習三:奇數或偶數
設計一函數可以輸入一個整數,函數回傳數值 1 表示是奇數,回傳數值 0 表示偶數。
Exercise 3: Odd or Even?
Design a function that takes one integer. It will return 1 if the integer is odd, return 0 if the integer is even.
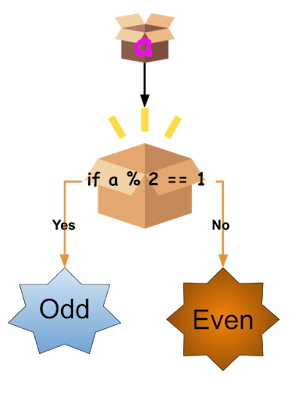
練習三參考解法:
Exercise 3 solution:
Design a function that takes one integer. It will return 1 if the integer is odd, return 0 if the integer is even.
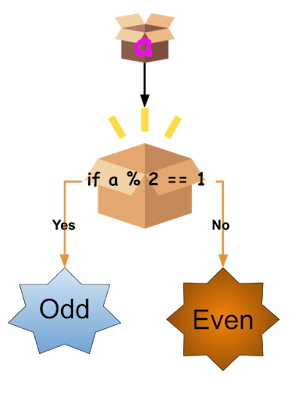
練習三參考解法:
Exercise 3 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | #include <stdio.h> #include <stdlib.h> int isOdd(int x) { // We can use return x % 2; return x % 2; // if( x % 2 == 1 ) return 1; // if( x % 2 == 0 ) return 0; } int main() { int x = 3; if( isOdd(x) == 1 ) printf("%d is odd.\n", x); else if( isOdd(x) == 0 ) printf("%d is even.\n", x); x = 4; if( isOdd(x) == 1 ) printf("%d is odd.\n", x); else if( isOdd(x) == 0 ) printf("%d is even.\n", x); return 0; } |
練習四:歡迎訊息
設計一個可以輸入兩個參數 n 與 msg 的函數,此函數用來輸出 msg 訊息 n 次。
Exercise 4: Greetings
Design a function that takes two parameters n and msg. This function will print msg n times.
練習四參考解法:
Exercise 4 solution:
練習五:互換
設計可以交換兩個整數的函數。
練習四參考解法:
Exercise 4 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> #include <stdlib.h> void prtMsg(char *msg, int n) { for(int i = 0; i < n; i++) printf("%s", msg); } int main() { int n = 3; char *msg = "Hello, player!\n"; prtMsg(msg, n); return 0; } |
練習五:互換
設計可以交換兩個整數的函數。
Exercise 5: Swap
Design a function that swap two integers.
練習五參考解法:
Exercise 5 solution:
練習六:二進位數字
設計一個可以將十進位數字轉換成二進位數字的函數。
Design a function that swap two integers.
練習五參考解法:
Exercise 5 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | #include <stdio.h> #include <stdlib.h> void swap(int *a, int *b) { // Method 1 int t = *a; *a = *b; *b = t; // Method 2 /* *a = *a + *b; *b = *a - *b; *a = *a - *b; */ } int main() { int i = 7, j = 8; printf("i = %d, j = %d\n", i, j); swap(&i, &j); printf("i = %d, j = %d\n", i, j); return 0; } |
練習六:二進位數字
設計一個可以將十進位數字轉換成二進位數字的函數。
Exercise 6: Binary Number
Design a function that converts a decimal number to a binary number.
練習六參考解法:
Exercise 6 solution:
Design a function that converts a decimal number to a binary number.
練習六參考解法:
Exercise 6 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | #include <stdio.h> #include <stdlib.h> void dToB(int d) { char b[32]; short p = 0; if(d == 0) printf("0"); while(d > 0) { b[p] = d % 2? '1':'0'; d = d / 2; p++; } while(--p >= 0) printf("%c", b[p]); printf("\t"); } int main() { for(int i = 0; i < 64; i++) dToB(i); return 0; } |
練習七:陣列中最大值
撰寫可以找出陣列中最大值的函數。
撰寫可以找出陣列中最大值的函數。
Exercise 7: Maximum in an array
Design a function that finds the maximum in an array.
練習七參考解法:
Exercise 7 solution:
Design a function that finds the maximum in an array.
練習七參考解法:
Exercise 7 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | /* Find the maximum in an array. Author: Holan */ #include <stdio.h> #include <stdlib.h> double getMax(double numbers[], int size) { // the maximum number double m = -1e9; for(int i = 0; i < size; i++) if(numbers[i] > m) m = numbers[i]; return m; } int main() { double data[] = {1, 3.23, 333, -334.5, 363}; int size = sizeof(data) / sizeof(double); printf("Max:%.2lf\n", getMax(data, size)); return 0; } |
練習八:總和
設計可以加總陣列中所有數值的函數。
設計可以加總陣列中所有數值的函數。
Exercise 8: Sum
Design a function that find sum of all elements in an array.
練習八參考解法:
Exercise 8 solution:
Design a function that find sum of all elements in an array.
練習八參考解法:
Exercise 8 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | /* Find sum of all elements in an array Author: Holan */ #include <stdio.h> #include <stdlib.h> double getSum(double numbers[], int size) { // The total double total = 0; for(int i = 0; i < size; i++) total += numbers[i]; return total; } int main() { double data[] = {1, 3.23, 333, -334.5, 363}; int size = sizeof(data) / sizeof(double); printf("Max:%.2lf\n", getSum(data, size)); return 0; } |
練習九:第幾象限
設計一個可以判斷(x, y)座標在第幾象限的函數。
Exercise 9: Coordinate
練習九參考解法:
Exercise 9 solution:
Design a function that prints the quadrant of the given point(x, y).
練習九參考解法:
Exercise 9 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | /* Prints the quadrant of the given point(x, y). Author: Holan */ #include <stdio.h> #include <stdlib.h> void getQuadrant(double x, double y) { if(x > 0 && y > 0) { printf("First"); } else if(x < 0 && y > 0) { printf("Second"); } else if(x < 0 && y < 0) { printf("Third"); } else if(x > 0 && y < 0) { printf("Fourth"); } printf(" Quadrant.\n"); } int main() { double x = 1.0, y = 1.0; getQuadrant(x,y); x = -1.0; getQuadrant(x,y); y = -1.0; getQuadrant(x,y); x = 1.0; getQuadrant(x,y); |
練習十:費氏數
設計一個可以算出第 N個費氏數的函數。
設計一個可以算出第 N個費氏數的函數。
Exercise 10:
Design a function that find the n-th Fibonacci number.
練習十參考解法:
Exercise 10 solution:
Design a function that find the n-th Fibonacci number.
練習十參考解法:
Exercise 10 solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | /* Find the n-th Fibonacci number. Author: Holan */ #include <stdio.h> #include <stdlib.h> int getFib(int N) { int fibo[10000]; fibo[0] = 0; fibo[1] = 1; for(int i = 2; i < N; i++) { fibo[i] = fibo[i-1] + fibo[i-2]; } return fibo[N-1]; } int main() { const int N = 10; for(int i = 1; i <= N; i++) printf("%2d ", getFib(i)); return 0; } |
沒有留言:
張貼留言